問題~コレクションフレームワーク~
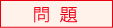
表示例と下記の用件を参考に、プログラムを作成しなさい。
■洋服クラス:Clothe
○フィールド
フィールド名 | name |
修飾子 | private |
static | なし |
final | なし |
型 | String |
説明 | 名前 |
○コンストラクタ
修飾子 | public |
戻り値 | なし |
static | なし |
引数 | String name |
処理 | 名前を設定 |
○メソッド
メソッド名 | getName |
修飾子 | public |
static | なし |
引数 | なし |
戻り値 | String 名前 |
処理 | 名前を取得する |
○フィールド
フィールド名 | clothesBoxList |
修飾子 | private |
static | なし |
final | なし |
型 | ArrayList |
説明 | 洋服ボックスリスト |
○コンストラクタ
修飾子 | public |
戻り値 | なし |
static | なし |
引数 | String name |
処理 | フィールドを初期化 |
○メソッド
メソッド名 | setClothesBox |
修飾子 | public |
static | なし |
引数 | ClothesBox box |
戻り値 | なし |
処理 | 洋服ボックスをフィールドに格納 |
メソッド名 | show |
修飾子 | public |
static | なし |
引数 | なし |
戻り値 | なし |
処理 | たんす内の情報を全て表示 |
■洋服ボックスクラス:ClothesBox
○フィールド
フィールド名 | clothList |
修飾子 | private |
static | なし |
final | なし |
型 | ArrayList |
説明 | 服リスト |
修飾子 | public |
戻り値 | なし |
static | なし |
引数 | なし |
処理 | フィールドを初期化 |
○メソッド
メソッド名 | setClothe |
修飾子 | public |
static | なし |
引数 | Clothe clothe |
戻り値 | なし |
処理 | 洋服をリストに格納 |
メソッド名 | getClothe |
修飾子 | public |
static | なし |
引数 | なし |
戻り値 | なし |
処理 | 洋服リストを取得 |
■メインクラス:Main
処理 |
① 下記の洋服を作成 レザージャケット ダウンジャケット 毛皮のジャケット ダンボールジャケット ステテコパンツ ステテコジャケット ② ①で作成した洋服を、洋服ボックスに設定する。 ③ ②で作成したボックスをたんすに設定する。 ④ たんすの中身を表示する。 |
回答
/** * 洋服クラス * @author RJC Human Resources */ public class Clothe { //名前 private String name = null; /** * コンストラクタ * @param name 名前 */ public Clothe ( String name) { //名前を設定 this.name = name; } /** * 名前を取得 * @return String 名前 */ public String getName(){ return name; } } import java.util.ArrayList; /** * 闘技場クラス * @author RJC Human Resources */ public class ClothesBox { //服リスト private ArrayList clothList; /** * コンストラクタ
* フィールドを初期化 */ public ClothesBox(){ this.clothList = new ArrayList(); } /** * 洋服をリストに格納 * @param clothe 洋服 */ public void setClothe(Clothe clothe){ //洋服を追加 this.clothList.add(clothe); } /** * 洋服リストを取得 * @return 洋服リスト */ public ArrayList getClothe(){ return this.clothList; } } import java.util.ArrayList; /* Lv8追加問題:問5解答 RJC Human Resource */ /** * たんすクラス * @author RJC HumanResource */ public class Commode { /** 洋服ボックスリスト */ private ArrayList clothesBoxList; /** * コンストラクタ
* フィールドを初期化 */ public Commode(){ this.clothesBoxList = new ArrayList(); } /** * 洋服ボックスをフィールドに格納 * @param box 洋服ボックス */ public void setClothesBox(ClothesBox box){ this.clothesBoxList.add(box); } /** * たんす内の情報を全て表示 */ public void show(){ System.out.println("===== 洋服一覧 ====="); //ボックスリスト分ループ for(int i = 0; i < this.clothesBoxList.size(); i++){ //ボックスを取得 ClothesBox box = (ClothesBox)this.clothesBoxList.get(i); //洋服リストを取得 ArrayList list = box.getClothe(); //洋服リスト分ループ for(int j = 0; j < list.size(); j++){ //洋服を取得 Clothe clothe = (Clothe)list.get(j); System.out.println(clothe.getName()); } } System.out.println("===================="); } } import java.util.ArrayList; /* Lv8追加問題:問4解答 RJC Human Resource */ /** * Mainクラス * @author RJC Human Resources */ public class Main { public static void main(String[] args) { //洋服を作成 Clothe clotheA = new Clothe("レザージャケット"); Clothe clotheB = new Clothe("ダウンジャケット"); Clothe clotheC = new Clothe("毛皮のジャケット"); Clothe clotheD = new Clothe("ダンボールジャケット"); Clothe clotheE = new Clothe("ステテコパンツ"); Clothe clotheF = new Clothe("ステテコジャケット"); //洋服をボックスに格納 ClothesBox box = new ClothesBox(); box.setClothe(clotheA); box.setClothe(clotheB); box.setClothe(clotheC); box.setClothe(clotheD); box.setClothe(clotheE); box.setClothe(clotheF); //ボックスをたんすに格納 Commode com = new Commode(); com.setClothesBox(box); //たんすの中身を表示 com.show(); } }