問題集~プロパティーファイル入出力~
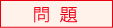
プロパティファイルから、年と月と何ヶ月分表示するのかを取得し、カレンダーを表示しなさい
【例】
プロパティファイルに下記の値が設定されている場合の処理
year=2008
month=10
count=3
【実行結果】
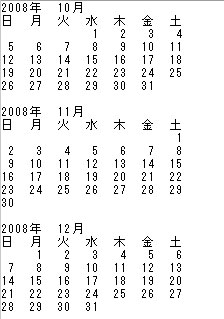
回答
import rjc.co.jp.sub.Calendar_04Sub; import rjc.co.jp.sub.Prop; public class Calendar_04Main{ public static void main(String args[]){ //プロパティファイルを読み込むサブクラスのインスタンス生成 Prop prop = new Prop(); //ファイルの読み込み prop.readProp("prop.properties"); //ビジネスクラスのインスタンス生成 Calendar_04Sub sub = new Calendar_04Sub(); //プロパティファイルに書き込まれているcount分ループ for(int i = 0; i < Integer.parseInt(prop.getCount()); i++){ //Showメソッド呼出し(ファイル内の値を設定 sub.show(Integer.parseInt(prop.getYear()), Integer.parseInt(prop.getMonth()) + i); //改行 System.out.println(); //改行 System.out.println(); } } } import java.util.GregorianCalendar; import java.util.Calendar; /** * GregorianCalendarクラスを利用して、カレンダーを表示します。 */ public class Calendar_04Sub{ /** * GregorianCalendarに引数をセットし、カレンダーを表示します。 * * @param year 年 * @param month 月 */ public void show(int year, int month){ //インスタンスの生成 GregorianCalendar cal = new GregorianCalendar(); //日付をセット cal.set(year, month - 1, 1); //引数の月の最後の日を決定 int max = cal.getActualMaximum(Calendar.DATE); //曜日を取得 int week = cal.get(Calendar.DAY_OF_WEEK); //年を取得 int yyyy = cal.get(Calendar.YEAR); int mm = cal.get(Calendar.MONTH)+1; //表示 System.out.println(yyyy + "年 " + mm + "月"); System.out.println("日 月 火 水 木 金 土"); //空白の表示 for (int i = 1; i < week;i++){ System.out.print(" "); } //土曜で改行するためのカウンタ int cun = week; //日付の表示 for( int i = 1; i <= max; i++){ //一桁の日付対応 if ( i <= 9){ System.out.print(" " + i + " "); } else { System.out.print(i + " "); } //土曜日になったら改行 if( cun % 7 == 0){ System.out.print("\n"); } cun++; } } } import java.io.FileInputStream; import java.util.Properties; public class Prop { /** 年 */ private String year; /** 月 */ private String month; /** 何か月か? */ private String count; /** * 引数で指定されたプロパティファイルを読み込む * @param fileName */ public void readProp(String fileName){ try{ //インスタンス生成 Properties prop = new Properties(); //ファイルの読み込み prop.load(new FileInputStream(fileName)); //年を取得 this.year = prop.getProperty("year"); //月を取得 this.month = prop.getProperty("month"); //日を取得 this.count = prop.getProperty("count"); }catch(Exception e){ e.printStackTrace(); } } /** * 年を取得 * @return String */ public String getYear(){ return this.year; } /** * 月を取得 * @return String */ public String getMonth(){ return this.month; } /** * カウントを取得 * @return String */ public String getCount(){ return this.count; } }